프로젝트에서 Typescript를 사용하다보면 가끔 type definition이 제공되지 않아 라이브러리를 쓰지 못하는 경우가 있다.
이 때 해결하는 방법이 두 가지 있다.
1. 로컬 솔루션: 로컬에 해당 폴더와 파일을 생성하기 (내 컴퓨터에서만 동작)
2. 궁극 솔루션: DefinitelyTyped 리포지토리에 PR을 신청하기 (다른 컴퓨터에서도 동작)
1번에 대해 한국어로 검색하면 정보가 나왔지만 2번의 경우엔 정보가 많지 않아 여기에 남겨본다.
1번도 2번에 포함되어 있기 때문에 1번을 먼저 설명하고 2번으로 넘어가겠다.
나의 경우엔 lucene-query-string-builder라는 라이브러리를 위한 type definition을 만드는 작업이었다.
로컬 솔루션
내가 사용하는 프로젝트의 로컬 폴더 중 node_modules의 @types 폴더 내에 아래와 같은 구조로 폴더와 파일을 생성하였다.
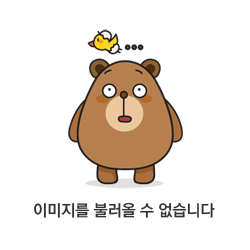
index.d.ts는 활용할 LuceneQueryStringBuilder 라이브러리의 index.js에 맞게 인터페이스를 만들었다.
export interface LuceneQueryStringBuilder {
/* ...... */
/**
* Combines queries with the OR operator.
* @param left - The queries to combine from left.
* @param right - The queries to combine from right.
*/
or(left: string, right: string): string;
/**
* Combines queries with the AND operator.
* @param left - The queries to combine from left.
* @param right - The queries to combine from right.
*/
and(left: string, right: string): string;
}
export const lucene: LuceneQueryStringBuilder;
사용은 import { lucene } from "lucene-query-string-builder"; 로 가져오면 된다.
여기까지만 해도 컴파일을 하면 type이 인식되면서 더이상 오류가 나지 않게 될 것이다.
궁극 솔루션
로컬 솔루션은 궁극적으로 외부와의 공유 시 의미가 없는 솔루션이기 때문에 DefinitelyTyped 리포에 pull request로 등록을 해야 한다.
DefinitelyTyped 리포지토리는 TypeScript 프로젝트에서 JavaScript 라이브러리를 사용할 때 타입 정의를 제공하는 오픈소스 커뮤니티 기반 저장소이다.
Pull requests 창만 봐도 얼마나 개발이 활발하게 진행되는 리포지토리인지 알 수 있다.
https://github.com/DefinitelyTyped/DefinitelyTyped/pulls
그만큼 올리는 과정 자체가 굉장히 까다롭고 명확하게 명시되어 있다.
영어: https://github.com/DefinitelyTyped/DefinitelyTyped/blob/master/README.md
한국어: https://github.com/DefinitelyTyped/DefinitelyTyped/blob/master/README.ko.md
위의 가이드라인을 모두 읽었다는 가정 하에 내가 진행했던 과정을 따라가보자.
먼저 DefinitelyTyped 리포를 Fork한다. https://github.com/DefinitelyTyped/DefinitelyTyped
GitHub - DefinitelyTyped/DefinitelyTyped: The repository for high quality TypeScript type definitions.
The repository for high quality TypeScript type definitions. - DefinitelyTyped/DefinitelyTyped
github.com
types에 내가 등록할 라이브러리 이름의 폴더를 생성하고 아래와 같은 파일 이름을 생성한다.
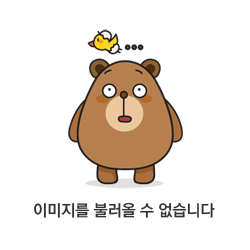
각 파일에 대한 설명은 위의 가이드라인을 참조하는 것도 좋고 types 내의 다른 프로젝트의 파일들을 참조하는 것도 좋다.
나는 후반부로 갈수록 후자의 도움을 많이 받았다.
이제 빌드 및 테스트를 해야할 시간인데 이 코드를 다 pnpm install을 하면 시간과 용량의 낭비이기 때문에 아래 형식으로 내가 필요한 라이브러리(lucene-query-string-builder)만 설치했다. (물론 공식 가이드라인에 설명이 있음)
pnpm install -w --filter "{./types/lucene-query-string-builder}"
그 후에 아래의 커멘드를 통해 테스트를 수행했다.
pnpm test lucene-query-string-builder
테스트 코드(lucene-query-string-builder-tests.ts)는 정말 정말 최소화시켰다.
import { lucene } from "lucene-query-string-builder";
lucene.term('hello'); //$ExpectType string
아마 처음에 오류가 많이 보일 것이다. 이 때부터 방망이를 깎는다는 심정으로 열심히 오류를 깎아 나가야 한다.
테스트가 문제 없이 끝나면 PR이 다 준비된 것이다.
나의 리포지토리에서 DefinitelyTyped 리포지토리 master로 PR을 생성하면, 나와 같이 신규로 등록하는 경우 아래의 11가지 조건을 다 만족해야 한다.
Please fill in this template.
- Use a meaningful title for the pull request.
- Include the name of the package modified.
- Test the change in your own code. (Compile and run.)
- Add or edit tests to reflect the change.
- Follow the advice from the readme.
- Avoid common mistakes.
- Run pnpm test <package to test>.
If adding a new definition:
- The package does not already provide its own types, or cannot have its .d.ts files generated via --declaration
- If this is for an npm package, match the name. If not, do not conflict with the name of an npm package.
- Create it with dts-gen --dt, not by basing it on an existing project.
- Represents shape of module/library correctly
- tsconfig.json should have noImplicitAny, noImplicitThis, strictNullChecks, and strictFunctionTypes set to true.
이 PR을 등록하면 CI/CD를 통해 자동으로 위 조건에 부합하는지 테스트가 수행된다. (너무 멋있다!)
나의 경우엔 중간에 문제가 있어 다시 커밋을 해야했지만, 그래도 결국 모든 자동 테스트를 통과했다.
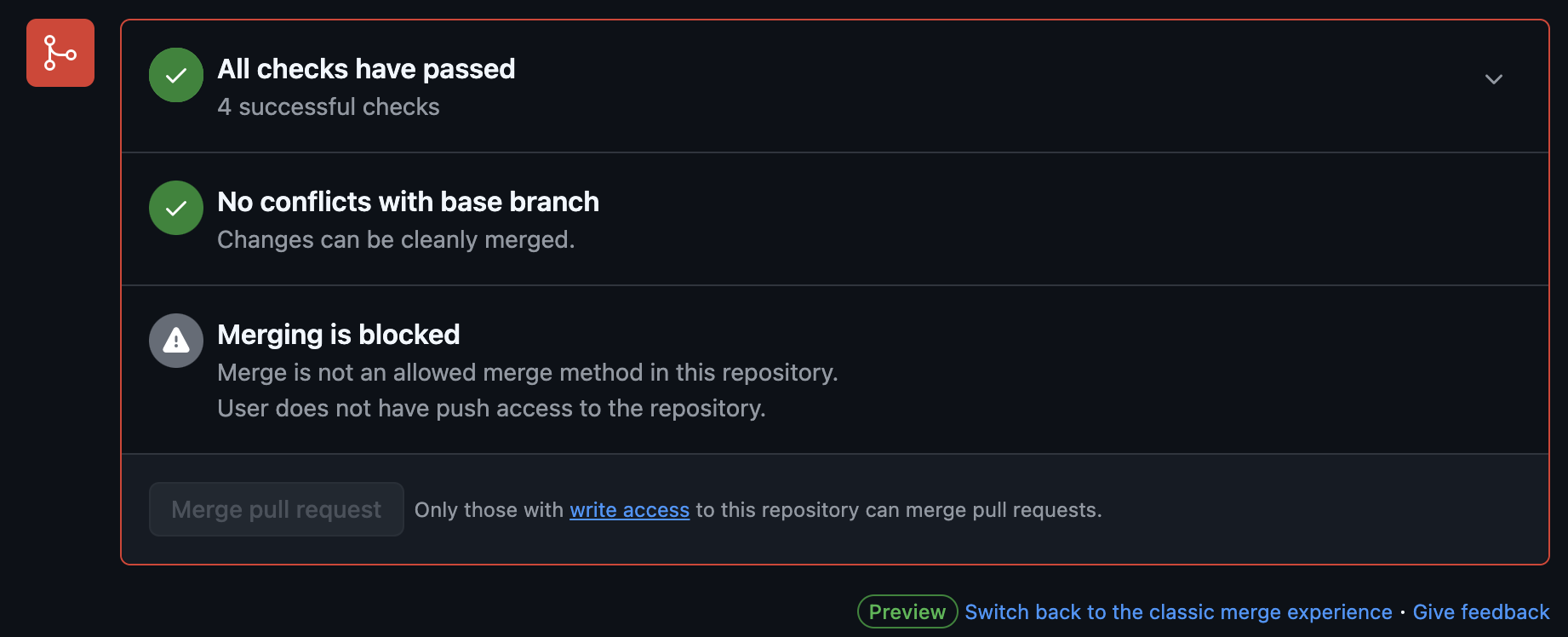
https://github.com/DefinitelyTyped/DefinitelyTyped/pull/71732
아직은 등록이 되지 않았기에 궁극 솔루션 성공을 외칠순 없지만, 그럼에도 이 경험은 공유되면 좋을 것 같아 끄적여본다.
부디 아무 문제 없이 조속하게 등록이 되길 바란다.
[[추가]]
리뷰를 위한 포맷팅을 위해 아래의 커멘드도 실행을 해야 한다.
pnpm dprint fmt
[[추추가]]
리뷰가 왔다! 내가 자바스크립트 원 라이브러리의 구현 방식을 따르지 않았다는 커멘트였다.
커멘트를 반영하고 테스트, 포맷팅을 한 후 커밋을 했다.
리뷰어가 이렇게 꼼꼼하게 리뷰를 해준다는 것에 놀라고 시간을 내준다는 것에 고마운 마음이 가득이다.
https://github.com/DefinitelyTyped/DefinitelyTyped/pull/71732#discussion_r1931365078
Add type definitions for lucene-query-string-builder by JinkiJung · Pull Request #71732 · DefinitelyTyped/DefinitelyTyped
Please fill in this template. Use a meaningful title for the pull request. Include the name of the package modified. Test the change in your own code. (Compile and run.) Add or edit tests to re...
github.com
[[추추추가]]
여러 번의 리뷰 끝에 드디어 merge 되었다!
https://github.com/DefinitelyTyped/DefinitelyTyped/commit/84367019576a54ae6fe758a50d956d5ed7f320aa
🤖 Merge PR #71732 Add type definitions for lucene-query-string-builde… · DefinitelyTyped/DefinitelyTyped@8436701
…r by @JinkiJung Co-authored-by: Jake Bailey <5341706+jakebailey@users.noreply.github.com>
github.com
이제 아래와 같이 설치가 가능하다.
pnpm i --save-dev @types/lucene-query-string-builder
npm 패키지까지 자동으로 배포되었다! 아쉽게도 아직은 검색 결과로 나오진 않는다.
https://www.npmjs.com/package/@types/lucene-query-string-builder
@types/lucene-query-string-builder
TypeScript definitions for lucene-query-string-builder. Latest version: 1.0.0, last published: 15 minutes ago. Start using @types/lucene-query-string-builder in your project by running `npm i @types/lucene-query-string-builder`. There are no other projects
www.npmjs.com
자동화 된 단계들이 너무 신기했다. 시간이 되면 리포지토리를 리뷰해봐야지.
'개발자의 인생' 카테고리의 다른 글
국제 표준 문서 저자가 되다 (0) | 2025.04.03 |
---|---|
연결 역할을 하는 생성형 인공지능 (1) | 2025.01.17 |
손으로 그린 그림을 3D 모델로 - Sketchurizer (0) | 2025.01.08 |
징징대는 것이 의외로 몸값을 올리는 이유 (1) | 2025.01.04 |
생활 속 모듈화와 분리가 개발자에게 중요한 이유 (1) | 2024.09.15 |